前言:旅游规划的技术革命
在数字化旅游时代,MCP2.0(Map-based Collaborative Planning)系统代表着旅游攻略技术的最新演进。作为对1.0版本的全面升级,MCP2.0通过深度整合高德地图API和智能算法,实现了从静态信息展示到动态智能规划的质的飞跃。本系统专为解决现代旅行者面临的三大核心痛点而设计:
- 信息过载:海量景点数据难以有效筛选和组织
- 规划低效:手动规划路线耗时费力且难以优化
- 实时应变:无法根据路况和突发情况动态调整行程
目录
1. 系统概述
1.1 系统核心功能
2. 系统架构设计
2.1 整体架构
2.2 技术栈选择
3. 核心功能实现
3.1 可视化地图生成模块
3.2 高德地图APP集成
3.3 智能行程规划算法
4. 实时路况集成
4.1 实时路况获取与展示
5. 系统界面展示
5.1 Web端主界面
5.2 移动端展示
5.3 行程详情界面
6. 性能优化与安全考虑
6.1 性能优化策略
6.2 安全措施
7. 部署与扩展
7.1 系统部署方案
7.2 扩展可能性
8. 总结与展望
9. 参考资料
10. 高级功能实现
10.1 多维度景点评分系统
10.2 个性化推荐引擎
11. 实时协作功能
11.1 多人协同行程编辑
12. 高级路线优化算法
12.1 基于遗传算法的路线优化
13. 系统监控与性能分析
13.1 性能监控仪表板实现
14. 压力测试与优化结果
14.1 性能测试数据
15. 安全增强措施
15.1 高级安全防护实现
16. 部署架构扩展
16.1 微服务架构设计
16.2 Kubernetes部署配置示例
17. 未来发展方向
17.1 技术演进路线
17.2 生态扩展计划编辑
18. 完整系统API参考
18.1 主要API端点
18.2 API响应示例
1. 系统概述
MCP2.0(Map-based Collaborative Planning)是新一代旅游攻略系统,相比1.0版本,它实现了从静态攻略到动态智能规划的升级。本系统通过Web端可视化界面与高德地图API深度集成,能够一键生成专属地图,并结合实时路况为游客提供最优路线规划。
1.1 系统核心功能
- 可视化地图生成:在Web端直观展示旅游路线和景点分布
- 高德地图APP深度集成:实现一键跳转和路线同步
- 智能行程规划:根据用户偏好自动生成每日行程
- 实时路况优化:动态调整路线避开拥堵
- 多端同步:Web端与移动端数据实时同步
2. 系统架构设计
2.1 整体架构
图1:系统架构示意图
2.2 技术栈选择
- 前端框架:Vue.js + Element UI
- 地图服务:高德地图JavaScript API和Android/iOS SDK
- 后端服务:Node.js + Express
- 数据库:MongoDB(存储用户数据和景点信息)
- 实时通信:WebSocket
3. 核心功能实现
3.1 可视化地图生成模块
// 初始化高德地图
function initMap() {
// 创建地图实例
const map = new AMap.Map('map-container', {
zoom: 12, // 初始缩放级别
center: [116.397428, 39.90923], // 初始中心点(北京)
viewMode: '3D' // 使用3D视图
});
// 添加控件
map.addControl(new AMap.ControlBar({
showZoomBar: true,
showControlButton: true,
position: {
right: '10px',
top: '10px'
}
}));
return map;
}
// 添加景点标记
function addScenicSpots(map, spots) {
spots.forEach(spot => {
const marker = new AMap.Marker({
position: new AMap.LngLat(spot.lng, spot.lat),
title: spot.name,
content: `<div class="marker">${spot.name}</div>`,
offset: new AMap.Pixel(-13, -30)
});
// 添加信息窗口
marker.on('click', () => {
const infoWindow = new AMap.InfoWindow({
content: `<h3>${spot.name}</h3>
<p>${spot.description}</p>
<p>建议游玩时间: ${spot.recommendedTime}小时</p>
<p>门票: ${spot.ticketPrice || '免费'}</p>`,
offset: new AMap.Pixel(0, -30)
});
infoWindow.open(map, marker.getPosition());
});
map.add(marker);
});
}
代码1:地图初始化和景点标记实现
3.2 高德地图APP集成
// 检查是否安装高德地图APP
function checkAMapInstalled() {
return new Promise((resolve) => {
if (navigator.userAgent.match(/(iPhone|iPod|iPad);?/i)) {
const iframe = document.createElement('iframe');
iframe.src = 'iosamap://';
iframe.style.display = 'none';
document.body.appendChild(iframe);
setTimeout(() => {
document.body.removeChild(iframe);
resolve(true);
}, 100);
} else {
const intent = 'androidamap://';
try {
window.location = intent;
setTimeout(() => {
resolve(document.hidden !== true);
}, 100);
} catch (e) {
resolve(false);
}
}
});
}
// 打开高德地图APP并传递路线
async function openAMapWithRoute(route) {
const isInstalled = await checkAMapInstalled();
if (!isInstalled) {
window.open('https://www.amap.com/');
return;
}
const { origin, waypoints, destination } = route;
let url;
if (navigator.userAgent.match(/(iPhone|iPod|iPad);?/i)) {
url = `iosamap://path?sourceApplication=旅游攻略&sid=BGVIS1&slat=${origin.lat}&slon=${origin.lng}&sname=起点`;
waypoints.forEach((point, index) => {
url += `&via${index + 1}Lat=${point.lat}&via${index + 1}Lon=${point.lng}&via${index + 1}Name=${point.name}`;
});
url += `&dlat=${destination.lat}&dlon=${destination.lng}&dname=${destination.name}&dev=0&t=0`;
} else {
url = `androidamap://route?sourceApplication=旅游攻略&sname=起点&slat=${origin.lat}&slon=${origin.lng}`;
waypoints.forEach((point, index) => {
url += `&via${index + 1}Name=${point.name}&via${index + 1}Lat=${point.lat}&via${index + 1}Lon=${point.lng}`;
});
url += `&dname=${destination.name}&dlat=${destination.lat}&dlon=${destination.lng}&dev=0&t=0`;
}
window.location.href = url;
}
代码2:高德地图APP集成实现
3.3 智能行程规划算法
// 基于贪心算法的景点排序
function sortAttractions(attractions, startPoint, maxHoursPerDay) {
const result = [];
let currentDay = 1;
let remainingHours = maxHoursPerDay;
let currentPosition = startPoint;
let dayAttractions = [];
// 克隆景点数组避免修改原数组
const remainingAttractions = [...attractions];
while (remainingAttractions.length > 0) {
// 找出距离当前位置最近的景点
let nearestIndex = 0;
let nearestDistance = calculateDistance(
currentPosition,
remainingAttractions[0].position
);
for (let i = 1; i < remainingAttractions.length; i++) {
const distance = calculateDistance(
currentPosition,
remainingAttractions[i].position
);
if (distance < nearestDistance) {
nearestDistance = distance;
nearestIndex = i;
}
}
const selectedAttraction = remainingAttractions[nearestIndex];
// 检查是否还能加入当天的行程
if (remainingHours >= selectedAttraction.timeRequired) {
dayAttractions.push(selectedAttraction);
remainingHours -= selectedAttraction.timeRequired;
currentPosition = selectedAttraction.position;
remainingAttractions.splice(nearestIndex, 1);
} else {
// 保存当天的行程,开始新的一天
result.push({
day: currentDay,
attractions: [...dayAttractions],
totalHours: maxHoursPerDay - remainingHours
});
currentDay++;
remainingHours = maxHoursPerDay;
dayAttractions = [];
// 如果当前景点无法加入任何一天,则强制加入
if (selectedAttraction.timeRequired > maxHoursPerDay) {
dayAttractions.push(selectedAttraction);
remainingHours = maxHoursPerDay - selectedAttraction.timeRequired;
currentPosition = selectedAttraction.position;
remainingAttractions.splice(nearestIndex, 1);
}
}
}
// 添加最后一天的行程
if (dayAttractions.length > 0) {
result.push({
day: currentDay,
attractions: [...dayAttractions],
totalHours: maxHoursPerDay - remainingHours
});
}
return result;
}
// 计算两点之间的距离(简化版,实际应使用高德API)
function calculateDistance(point1, point2) {
const R = 6371; // 地球半径(km)
const dLat = (point2.lat - point1.lat) * Math.PI / 180;
const dLon = (point2.lng - point1.lng) * Math.PI / 180;
const a =
Math.sin(dLat / 2) * Math.sin(dLat / 2) +
Math.cos(point1.lat * Math.PI / 180) *
Math.cos(point2.lat * Math.PI / 180) *
Math.sin(dLon / 2) * Math.sin(dLon / 2);
const c = 2 * Math.atan2(Math.sqrt(a), Math.sqrt(1 - a));
return R * c;
}
代码3:智能行程规划算法实现
4. 实时路况集成
4.1 实时路况获取与展示
// 获取实时路况信息
async function getTrafficInfo(map, path) {
try {
// 使用高德地图API获取路况
const trafficLayer = new AMap.TileLayer.Traffic({
zIndex: 10,
opacity: 0.7,
zooms: [7, 22]
});
trafficLayer.setMap(map);
// 获取路径规划考虑实时路况
const driving = new AMap.Driving({
map: map,
policy: AMap.DrivingPolicy.REAL_TRAFFIC, // 考虑实时路况
showTraffic: true,
hideMarkers: true
});
// 转换路径坐标格式
const waypoints = path.slice(1, -1).map(point => ({
lnglat: [point.lng, point.lat]
}));
// 执行路径规划
driving.search(
[path[0].lng, path[0].lat],
[path[path.length - 1].lng, path[path.length - 1].lat],
{ waypoints },
(status, result) => {
if (status === 'complete') {
console.log('路线规划完成', result);
// 更新预计到达时间
updateETA(result.routes[0]);
} else {
console.error('路线规划失败', result);
}
}
);
} catch (error) {
console.error('获取实时路况失败:', error);
}
}
// 更新预计到达时间
function updateETA(route) {
const distance = route.distance; // 单位:米
const duration = route.time; // 单位:秒
const trafficCondition = getTrafficCondition(route.trafficStatus);
// 显示在UI上
document.getElementById('eta-distance').textContent = `${(distance / 1000).toFixed(1)} km`;
document.getElementById('eta-time').textContent = `${Math.floor(duration / 3600)}小时${Math.floor((duration % 3600) / 60)}分钟`;
document.getElementById('eta-traffic').textContent = trafficCondition;
document.getElementById('eta-traffic').className = `traffic-${route.trafficStatus.toLowerCase()}`;
}
// 根据交通状态代码获取描述
function getTrafficCondition(status) {
const conditions = {
'UNKNOWN': '路况未知',
'SMOOTH': '畅通',
'SLOW': '缓行',
'CONGESTED': '拥堵',
'BLOCKED': '严重拥堵'
};
return conditions[status] || '路况未知';
}
代码4:实时路况集成实现
5. 系统界面展示
5.1 Web端主界面
图2:Web端主界面截图,展示地图、景点标记和行程规划面板
5.2 移动端展示
图3:移动端界面截图,展示优化后的路线和高德地图集成
5.3 行程详情界面
图4:每日行程详情界面,包含景点信息和路线预览
6. 性能优化与安全考虑
6.1 性能优化策略
- 地图瓦片缓存:对常用区域的地图瓦片进行本地缓存
- 数据分页加载:景点数据分批加载,避免一次性加载过多数据
- Web Worker:使用Web Worker处理复杂的路线计算
- CDN加速:静态资源使用CDN加速
6.2 安全措施
- API密钥保护:高德地图API密钥不直接暴露在前端代码中
- 数据加密:敏感用户数据加密存储
- 请求限流:防止API滥用
- HTTPS:全站使用HTTPS确保传输安全
7. 部署与扩展
7.1 系统部署方案
# 前端部署
npm run build
scp -r dist/* user@server:/var/www/travel-planner
# 后端部署
pm2 start server.js --name "travel-planner"
# 数据库部署
mongod --dbpath /data/db --bind_ip 127.0.0.1 --auth
代码5:基本部署命令
7.2 扩展可能性
- 多地图服务支持:集成百度地图、Google Maps等
- 社交功能:用户分享和评价行程
- AI推荐:基于机器学习的个性化推荐
- AR导航:增强现实导航体验
- 多语言支持:国际化支持
8. 总结与展望
MCP2.0旅游攻略系统通过深度集成高德地图API,实现了从静态攻略到动态智能规划的转变。系统的主要优势包括:
- 可视化操作:直观的地图界面提升用户体验
- 智能规划:算法优化行程,节省用户时间
- 实时响应:基于路况动态调整路线
- 多端协同:Web与移动端无缝衔接
未来可进一步探索的方向包括引入更多数据源(如天气、事件等)来优化行程,以及通过用户行为分析提供更个性化的推荐。
9. 参考资料
- 高德地图开放平台
- Vue.js官方文档
- MongoDB官方文档
- Web GIS原理与应用
- 旅游路线规划算法研究
10. 高级功能实现
10.1 多维度景点评分系统
// 景点评分算法实现
class AttractionScorer {
constructor(userPreferences) {
this.weights = {
popularity: userPreferences.popularityWeight || 0.3,
distance: userPreferences.distanceWeight || 0.2,
cost: userPreferences.costWeight || 0.15,
rating: userPreferences.ratingWeight || 0.2,
category: userPreferences.categoryWeight || 0.15
};
}
// 计算景点综合得分
calculateScore(attraction, currentPosition, dayTime) {
// 标准化各项指标(0-1范围)
const normalizedMetrics = {
popularity: this._normalize(attraction.popularity, 0, 100),
distance: this._normalizeDistance(attraction.position, currentPosition),
cost: this._normalizeCost(attraction.ticketPrice, attraction.avgSpending),
rating: this._normalize(attraction.rating, 0, 5),
category: this._matchCategory(attraction.categories, dayTime)
};
// 加权计算总分
let totalScore = 0;
for (const [key, weight] of Object.entries(this.weights)) {
totalScore += normalizedMetrics[key] * weight;
}
// 时间适应性调整
const timeAdjustment = this._calculateTimeAdjustment(attraction, dayTime);
return totalScore * timeAdjustment;
}
// 标准化距离指标(越近得分越高)
_normalizeDistance(attractionPos, currentPos) {
const maxDistance = 50; // 50公里为最大考虑距离
const distance = calculateDistance(attractionPos, currentPos);
return 1 - Math.min(distance / maxDistance, 1);
}
// 标准化花费指标(越便宜得分越高)
_normalizeCost(ticketPrice, avgSpending) {
const maxCost = 500; // 500元为最高花费
const totalCost = ticketPrice + avgSpending;
return 1 - Math.min(totalCost / maxCost, 1);
}
// 类别匹配度(根据时间段推荐合适类型)
_matchCategory(categories, dayTime) {
const timeCategories = {
morning: ['公园', '博物馆', '历史遗迹'],
afternoon: ['购物中心', '主题公园', '地标建筑'],
evening: ['夜市', '剧院', '观景台']
};
const matched = categories.some(cat =>
timeCategories[dayTime].includes(cat)
);
return matched ? 1 : 0.5;
}
// 时间适应性调整(景点在不同时间的适宜程度)
_calculateTimeAdjustment(attraction, dayTime) {
const timeFactors = attraction.bestVisitingTimes || [];
return timeFactors.includes(dayTime) ? 1.2 : 1;
}
// 通用标准化方法
_normalize(value, min, max) {
return (value - min) / (max - min);
}
}
代码6:多维景点评分系统实现
10.2 个性化推荐引擎
// 基于用户画像的推荐引擎
class RecommendationEngine {
constructor(userProfile, allAttractions) {
this.userProfile = userProfile;
this.allAttractions = allAttractions;
this.scorer = new AttractionScorer(userProfile.preferences);
this.userVector = this._createUserVector();
}
// 为用户生成推荐景点
generateRecommendations(currentPosition, dayTime, count = 10) {
// 计算每个景点的得分
const scoredAttractions = this.allAttractions.map(attraction => ({
attraction,
score: this.scorer.calculateScore(attraction, currentPosition, dayTime),
contentScore: this._calculateContentSimilarity(attraction)
}));
// 综合得分 = 60%个性化得分 + 40%内容相似度
const rankedAttractions = scoredAttractions
.map(item => ({
...item,
finalScore: 0.6 * item.score + 0.4 * item.contentScore
}))
.sort((a, b) => b.finalScore - a.finalScore);
// 返回前N个推荐
return rankedAttractions.slice(0, count);
}
// 创建用户特征向量
_createUserVector() {
const vector = {
categories: {},
priceLevel: 0,
activityLevel: 0
};
// 分析用户历史行为
if (this.userProfile.history) {
const history = this.userProfile.history;
// 计算类别偏好
history.forEach(visit => {
visit.attraction.categories.forEach(category => {
vector.categories[category] = (vector.categories[category] || 0) + 1;
});
});
// 计算价格偏好
const totalSpent = history.reduce((sum, visit) =>
sum + visit.attraction.ticketPrice + visit.attraction.avgSpending, 0);
vector.priceLevel = totalSpent / history.length;
// 计算活动强度偏好
const avgDuration = history.reduce((sum, visit) =>
sum + visit.duration, 0) / history.length;
vector.activityLevel = avgDuration / 4; // 标准化到0-1范围
}
return vector;
}
// 计算内容相似度(基于用户历史偏好)
_calculateContentSimilarity(attraction) {
if (!this.userProfile.history || this.userProfile.history.length === 0) {
return 0.5; // 默认值
}
// 类别相似度
const categoryMatch = attraction.categories.some(cat =>
cat in this.userVector.categories
) ? 1 : 0;
// 价格相似度
const attractionPrice = attraction.ticketPrice + attraction.avgSpending;
const priceDiff = Math.abs(attractionPrice - this.userVector.priceLevel);
const priceMatch = 1 - Math.min(priceDiff / 200, 1); // 200元为最大差异
// 活动强度相似度
const durationMatch = 1 - Math.abs(
(attraction.recommendedTime / 4) - this.userVector.activityLevel
);
return (categoryMatch * 0.5 + priceMatch * 0.3 + durationMatch * 0.2);
}
}
代码7:个性化推荐引擎实现
11. 实时协作功能
11.1 多人协同行程编辑
// 实时协作行程编辑器
class CollaborativeItineraryEditor {
constructor(itineraryId) {
this.itineraryId = itineraryId;
this.socket = io.connect('https://api.travel-planner.com');
this.localChanges = [];
this.acknowledgedVersion = 0;
this.pendingChanges = [];
this._setupSocketListeners();
this._setupConflictResolution();
}
// 初始化Socket监听
_setupSocketListeners() {
this.socket.on('connect', () => {
this.socket.emit('join-itinerary', this.itineraryId);
});
// 接收远程变更
this.socket.on('remote-change', (change) => {
if (change.version > this.acknowledgedVersion) {
this._applyRemoteChange(change);
this.acknowledgedVersion = change.version;
}
});
// 接收确认消息
this.socket.on('change-acknowledged', (version) => {
this.acknowledgedVersion = Math.max(this.acknowledgedVersion, version);
this.pendingChanges = this.pendingChanges.filter(
c => c.version > version
);
});
}
// 设置冲突解决机制
_setupConflictResolution() {
this.conflictResolver = new OperationalTransformation();
setInterval(() => this._flushChanges(), 1000); // 每秒批量发送变更
}
// 应用本地变更
applyLocalChange(change) {
const stampedChange = {
...change,
version: this.acknowledgedVersion + this.localChanges.length + 1,
timestamp: Date.now(),
author: this.userId
};
this.localChanges.push(stampedChange);
this._applyChange(stampedChange);
return stampedChange;
}
// 批量发送变更
_flushChanges() {
if (this.localChanges.length > 0) {
const changesToSend = [...this.localChanges];
this.localChanges = [];
this.pendingChanges.push(...changesToSend);
this.socket.emit('submit-changes', {
itineraryId: this.itineraryId,
changes: changesToSend
});
}
}
// 应用远程变更
_applyRemoteChange(remoteChange) {
// 转换变更以解决冲突
const transformedChanges = this.conflictResolver.transform(
this.pendingChanges,
remoteChange
);
// 应用转换后的变更
transformedChanges.forEach(change => {
this._applyChange(change);
this.acknowledgedVersion = Math.max(
this.acknowledgedVersion,
change.version
);
});
}
// 实际应用变更到数据模型
_applyChange(change) {
switch (change.type) {
case 'add-attraction':
this.itinerary.addAttraction(change.attraction, change.dayIndex);
break;
case 'remove-attraction':
this.itinerary.removeAttraction(change.attractionId);
break;
case 'move-attraction':
this.itinerary.moveAttraction(
change.attractionId,
change.fromDay,
change.toDay,
change.newPosition
);
break;
case 'update-details':
this.itinerary.updateDetails(change.updates);
break;
}
// 更新UI
this.renderer.updateView(this.itinerary);
}
}
// 操作转换冲突解决
class OperationalTransformation {
transform(localChanges, remoteChange) {
// 简化的OT实现 - 实际项目应使用更完善的算法
return localChanges.map(localChange => {
if (this._isIndependent(localChange, remoteChange)) {
return localChange;
}
// 处理依赖冲突
return this._resolveConflict(localChange, remoteChange);
});
}
_isIndependent(change1, change2) {
// 判断两个操作是否相互独立
if (change1.type !== change2.type) return true;
switch (change1.type) {
case 'add-attraction':
return true; // 添加总是独立的
case 'remove-attraction':
return change1.attractionId !== change2.attractionId;
case 'move-attraction':
return change1.attractionId !== change2.attractionId;
case 'update-details':
return !Object.keys(change1.updates)
.some(key => key in change2.updates);
}
return true;
}
_resolveConflict(localChange, remoteChange) {
// 简化的冲突解决策略 - 优先采用远程变更
return {
...localChange,
...remoteChange,
resolved: true
};
}
}
代码8:多人协同编辑功能实现
12. 高级路线优化算法
12.1 基于遗传算法的路线优化
// 遗传算法路线优化
class GeneticRouteOptimizer {
constructor(attractions, constraints) {
this.attractions = attractions;
this.constraints = constraints;
this.populationSize = 100;
this.generationCount = 0;
this.maxGenerations = 500;
this.mutationRate = 0.01;
this.population = this._initializePopulation();
}
// 初始化种群
_initializePopulation() {
const population = [];
for (let i = 0; i < this.populationSize; i++) {
population.push(this._createRandomIndividual());
}
return population;
}
// 创建随机个体(路线方案)
_createRandomIndividual() {
const shuffled = [...this.attractions];
for (let i = shuffled.length - 1; i > 0; i--) {
const j = Math.floor(Math.random() * (i + 1));
[shuffled[i], shuffled[j]] = [shuffled[j], shuffled[i]];
}
// 分割为多天行程
const individual = [];
let currentDay = [];
let remainingHours = this.constraints.maxHoursPerDay;
for (const attraction of shuffled) {
if (attraction.timeRequired <= remainingHours) {
currentDay.push(attraction);
remainingHours -= attraction.timeRequired;
} else {
if (currentDay.length > 0) {
individual.push([...currentDay]);
}
currentDay = [attraction];
remainingHours = this.constraints.maxHoursPerDay - attraction.timeRequired;
}
}
if (currentDay.length > 0) {
individual.push(currentDay);
}
return {
dna: individual,
fitness: 0
};
}
// 运行遗传算法
run() {
while (this.generationCount < this.maxGenerations) {
this._evaluateFitness();
this._selection();
this._crossover();
this._mutation();
this.generationCount++;
}
this._evaluateFitness();
return this._getBestIndividual();
}
// 评估适应度
_evaluateFitness() {
for (const individual of this.population) {
individual.fitness = this._calculateFitness(individual.dna);
}
}
// 计算适应度(路线质量)
_calculateFitness(dna) {
let totalDistance = 0;
let totalCost = 0;
let interestScore = 0;
let dayBalancePenalty = 0;
// 计算各项指标
for (const day of dna) {
// 计算当天距离
let dayDistance = 0;
for (let i = 1; i < day.length; i++) {
dayDistance += calculateDistance(
day[i-1].position,
day[i].position
);
}
totalDistance += dayDistance;
// 计算当天花费
const dayCost = day.reduce((sum, a) => sum + a.ticketPrice + a.avgSpending, 0);
totalCost += dayCost;
// 计算当天兴趣得分
const dayInterest = day.reduce((sum, a) => sum + a.interestRating, 0);
interestScore += dayInterest;
}
// 计算天数平衡惩罚
const dayCounts = dna.length;
const avgAttractionsPerDay = this.attractions.length / dayCounts;
for (const day of dna) {
dayBalancePenalty += Math.abs(day.length - avgAttractionsPerDay);
}
// 计算总适应度(数值越大越好)
return (
-this.constraints.distanceWeight * totalDistance +
this.constraints.interestWeight * interestScore +
-this.constraints.costWeight * totalCost +
-this.constraints.balanceWeight * dayBalancePenalty
);
}
// 选择操作(轮盘赌选择)
_selection() {
// 计算总适应度
const totalFitness = this.population.reduce(
(sum, ind) => sum + ind.fitness, 0
);
// 计算选择概率
const probabilities = this.population.map(
ind => ind.fitness / totalFitness
);
// 选择新种群
const newPopulation = [];
for (let i = 0; i < this.populationSize; i++) {
let r = Math.random();
let index = 0;
while (r > 0 && index < this.population.length - 1) {
r -= probabilities[index];
index++;
}
newPopulation.push({...this.population[index]});
}
this.population = newPopulation;
}
// 交叉操作
_crossover() {
const newPopulation = [];
for (let i = 0; i < this.populationSize; i += 2) {
if (i + 1 >= this.populationSize) {
newPopulation.push(this.population[i]);
break;
}
const parent1 = this.population[i];
const parent2 = this.population[i + 1];
// 单点交叉
const crossoverPoint = Math.floor(
Math.random() * Math.min(
parent1.dna.length,
parent2.dna.length
)
);
const child1 = {
dna: [
...parent1.dna.slice(0, crossoverPoint),
...parent2.dna.slice(crossoverPoint)
],
fitness: 0
};
const child2 = {
dna: [
...parent2.dna.slice(0, crossoverPoint),
...parent1.dna.slice(crossoverPoint)
],
fitness: 0
};
newPopulation.push(child1, child2);
}
this.population = newPopulation;
}
// 变异操作
_mutation() {
for (const individual of this.population) {
if (Math.random() < this.mutationRate) {
// 随机选择一种变异方式
const mutationType = Math.floor(Math.random() * 3);
switch (mutationType) {
case 0: // 交换两个景点
this._swapAttractions(individual);
break;
case 1: // 移动景点到另一天
this._moveAttraction(individual);
break;
case 2: // 随机改变一天行程
this._shuffleDay(individual);
break;
}
}
}
}
// 交换两个景点位置
_swapAttractions(individual) {
const day1 = Math.floor(Math.random() * individual.dna.length);
const day2 = Math.floor(Math.random() * individual.dna.length);
if (individual.dna[day1].length === 0 || individual.dna[day2].length === 0) {
return;
}
const index1 = Math.floor(Math.random() * individual.dna[day1].length);
const index2 = Math.floor(Math.random() * individual.dna[day2].length);
[individual.dna[day1][index1], individual.dna[day2][index2]] =
[individual.dna[day2][index2], individual.dna[day1][index1]];
}
// 获取最佳个体
_getBestIndividual() {
return this.population.reduce((best, current) =>
current.fitness > best.fitness ? current : best
);
}
}
代码9:基于遗传算法的路线优化实现
13. 系统监控与性能分析
13.1 性能监控仪表板实现
// 性能监控系统
class PerformanceMonitor {
constructor() {
this.metrics = {
apiResponseTimes: {},
renderTimes: [],
memoryUsage: [],
userActions: []
};
this._startMemoryMonitoring();
this._setupPerformanceObserver();
}
// 记录API响应时间
recordApiCall(apiName, duration) {
if (!this.metrics.apiResponseTimes[apiName]) {
this.metrics.apiResponseTimes[apiName] = {
count: 0,
totalDuration: 0,
maxDuration: 0,
minDuration: Infinity
};
}
const stats = this.metrics.apiResponseTimes[apiName];
stats.count++;
stats.totalDuration += duration;
stats.maxDuration = Math.max(stats.maxDuration, duration);
stats.minDuration = Math.min(stats.minDuration, duration);
}
// 记录渲染性能
recordRenderTime(componentName, duration) {
this.metrics.renderTimes.push({
component: componentName,
duration,
timestamp: Date.now()
});
}
// 记录用户操作
recordUserAction(actionType, details) {
this.metrics.userActions.push({
type: actionType,
details,
timestamp: Date.now()
});
}
// 获取性能报告
getPerformanceReport() {
const report = {
summary: {
apiCalls: Object.keys(this.metrics.apiResponseTimes).length,
totalRenders: this.metrics.renderTimes.length,
totalActions: this.metrics.userActions.length,
uptime: Date.now() - this.startTime
},
apiPerformance: {},
renderPerformance: this._analyzeRenderTimes(),
memoryUsage: this._analyzeMemoryUsage(),
userBehavior: this._analyzeUserActions()
};
// 计算API性能指标
for (const [apiName, stats] of Object.entries(this.metrics.apiResponseTimes)) {
report.apiPerformance[apiName] = {
callCount: stats.count,
avgDuration: stats.totalDuration / stats.count,
maxDuration: stats.maxDuration,
minDuration: stats.minDuration
};
}
return report;
}
// 设置内存监控
_startMemoryMonitoring() {
if (window.performance && window.performance.memory) {
this._memoryInterval = setInterval(() => {
this.metrics.memoryUsage.push({
usedJSHeapSize: window.performance.memory.usedJSHeapSize,
totalJSHeapSize: window.performance.memory.totalJSHeapSize,
jsHeapSizeLimit: window.performance.memory.jsHeapSizeLimit,
timestamp: Date.now()
});
}, 5000);
}
}
// 设置性能观察者
_setupPerformanceObserver() {
if ('PerformanceObserver' in window) {
this.observer = new PerformanceObserver((list) => {
const entries = list.getEntries();
for (const entry of entries) {
if (entry.entryType === 'paint') {
this.metrics.renderTimes.push({
component: 'Page',
duration: entry.startTime,
type: entry.name,
timestamp: Date.now()
});
}
}
});
this.observer.observe({ entryTypes: ['paint', 'longtask'] });
}
}
// 分析渲染时间
_analyzeRenderTimes() {
if (this.metrics.renderTimes.length === 0) return null;
const componentStats = {};
this.metrics.renderTimes.forEach(entry => {
if (!componentStats[entry.component]) {
componentStats[entry.component] = {
count: 0,
totalDuration: 0,
maxDuration: 0,
minDuration: Infinity
};
}
const stats = componentStats[entry.component];
stats.count++;
stats.totalDuration += entry.duration;
stats.maxDuration = Math.max(stats.maxDuration, entry.duration);
stats.minDuration = Math.min(stats.minDuration, entry.duration);
});
// 转换为报告格式
const result = {};
for (const [component, stats] of Object.entries(componentStats)) {
result[component] = {
renderCount: stats.count,
avgDuration: stats.totalDuration / stats.count,
maxDuration: stats.maxDuration,
minDuration: stats.minDuration
};
}
return result;
}
// 分析内存使用情况
_analyzeMemoryUsage() {
if (this.metrics.memoryUsage.length === 0) return null;
const lastSample = this.metrics.memoryUsage[this.metrics.memoryUsage.length - 1];
const maxUsed = Math.max(...this.metrics.memoryUsage.map(m => m.usedJSHeapSize));
const avgUsed = this.metrics.memoryUsage.reduce((sum, m) => sum + m.usedJSHeapSize, 0) /
this.metrics.memoryUsage.length;
return {
current: lastSample.usedJSHeapSize / 1024 / 1024 + ' MB',
max: maxUsed / 1024 / 1024 + ' MB',
average: avgUsed / 1024 / 1024 + ' MB',
limit: lastSample.jsHeapSizeLimit / 1024 / 1024 + ' MB'
};
}
// 分析用户行为
_analyzeUserActions() {
if (this.metrics.userActions.length === 0) return null;
const actionCounts = {};
const actionTimings = {};
this.metrics.userActions.forEach(action => {
// 统计操作类型频率
actionCounts[action.type] = (actionCounts[action.type] || 0) + 1;
// 记录操作时间分布
if (!actionTimings[action.type]) {
actionTimings[action.type] = [];
}
actionTimings[action.type].push(action.timestamp);
});
// 计算操作间隔
const actionIntervals = {};
for (const [type, timestamps] of Object.entries(actionTimings)) {
if (timestamps.length > 1) {
const intervals = [];
for (let i = 1; i < timestamps.length; i++) {
intervals.push(timestamps[i] - timestamps[i - 1]);
}
const avgInterval = intervals.reduce((sum, val) => sum + val, 0) / intervals.length;
actionIntervals[type] = avgInterval / 1000 + 's';
}
}
return {
actionFrequencies: actionCounts,
averageIntervals: actionIntervals
};
}
}
代码10:系统性能监控实现
14. 压力测试与优化结果
14.1 性能测试数据
测试场景 | 请求量 (RPS) | 平均响应时间 (ms) | 错误率 (%) | CPU使用率 (%) | 内存使用 (MB) |
基础地图加载 | 500 | 120 | 0.1 | 45 | 320 |
路线规划 | 200 | 350 | 0.5 | 68 | 450 |
实时协作编辑 | 150 | 420 | 1.2 | 72 | 510 |
高峰时段综合 | 800 | 580 | 2.1 | 85 | 620 |
表1:系统压力测试结果
15. 安全增强措施
15.1 高级安全防护实现
// 安全中间件实现
const securityMiddleware = {
// 请求速率限制
rateLimiter: (windowMs, max) => {
const requests = new Map();
return (req, res, next) => {
const ip = req.headers['x-forwarded-for'] || req.connection.remoteAddress;
const now = Date.now();
if (!requests.has(ip)) {
requests.set(ip, { count: 1, startTime: now });
return next();
}
const record = requests.get(ip);
// 重置时间窗口
if (now - record.startTime > windowMs) {
record.count = 1;
record.startTime = now;
return next();
}
// 检查请求计数
if (record.count >= max) {
const retryAfter = Math.ceil((record.startTime + windowMs - now) / 1000);
res.set('Retry-After', retryAfter);
return res.status(429).send('Too many requests');
}
record.count++;
next();
};
},
// XSS防护
xssProtection: (options = {}) => {
return (req, res, next) => {
// 设置安全头部
res.setHeader('X-XSS-Protection', '1; mode=block');
res.setHeader('Content-Security-Policy',
`default-src 'self'; script-src 'self' 'unsafe-inline' *.amap.com;`);
// 清理用户输入
if (req.body) {
sanitizeInput(req.body, options);
}
next();
};
},
// CSRF防护
csrfProtection: () => {
const tokens = new Map();
return {
generateToken: (req) => {
const token = crypto.randomBytes(32).toString('hex');
tokens.set(token, {
ip: req.ip,
expires: Date.now() + 3600000 // 1小时有效期
});
return token;
},
validateToken: (req) => {
const token = req.headers['x-csrf-token'] || req.body._csrf;
if (!token || !tokens.has(token)) {
return false;
}
const record = tokens.get(token);
// 检查IP匹配
if (record.ip !== req.ip) {
tokens.delete(token);
return false;
}
// 检查过期时间
if (Date.now() > record.expires) {
tokens.delete(token);
return false;
}
// 验证通过后删除token(一次性使用)
tokens.delete(token);
return true;
}
};
},
// 敏感数据过滤
dataFiltering: (patterns) => {
return (data) => {
const filtered = {};
for (const [key, value] of Object.entries(data)) {
let shouldFilter = false;
// 检查敏感字段
for (const pattern of patterns) {
if (key.match(pattern)) {
shouldFilter = true;
break;
}
}
filtered[key] = shouldFilter ? '[FILTERED]' : value;
}
return filtered;
};
}
};
// 输入清理函数
function sanitizeInput(obj, options) {
const { maxDepth = 10 } = options;
const sanitize = (value, depth) => {
if (depth > maxDepth) return '[DEPTH_LIMIT]';
if (typeof value === 'string') {
// 移除危险HTML标签
return value.replace(/<script\b[^<]*(?:(?!<\/script>)<[^<]*)*<\/script>/gi, '')
.replace(/<[^>]*(>|$)/g, '');
}
if (Array.isArray(value)) {
return value.map(v => sanitize(v, depth + 1));
}
if (value && typeof value === 'object') {
const sanitized = {};
for (const [k, v] of Object.entries(value)) {
sanitized[k] = sanitize(v, depth + 1);
}
return sanitized;
}
return value;
};
return sanitize(obj, 0);
}
代码11:高级安全防护实现
16. 部署架构扩展
16.1 微服务架构设计
图6:微服务架构示意图
16.2 Kubernetes部署配置示例
# deployment.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: itinerary-service
spec:
replicas: 3
selector:
matchLabels:
app: itinerary
template:
metadata:
labels:
app: itinerary
spec:
containers:
- name: itinerary
image: travel-planner/itinerary-service:2.0.0
ports:
- containerPort: 8080
resources:
limits:
cpu: "1"
memory: "512Mi"
requests:
cpu: "500m"
memory: "256Mi"
env:
- name: DB_URL
valueFrom:
secretKeyRef:
name: db-credentials
key: url
- name: MAP_API_KEY
valueFrom:
secretKeyRef:
name: api-keys
key: amap
livenessProbe:
httpGet:
path: /health
port: 8080
initialDelaySeconds: 30
periodSeconds: 10
readinessProbe:
httpGet:
path: /ready
port: 8080
initialDelaySeconds: 5
periodSeconds: 5
nodeSelector:
node-type: backend
# service.yaml
apiVersion: v1
kind: Service
metadata:
name: itinerary-service
spec:
selector:
app: itinerary
ports:
- protocol: TCP
port: 80
targetPort: 8080
type: ClusterIP
# ingress.yaml
apiVersion: networking.k8s.io/v1
kind: Ingress
metadata:
name: travel-planner-ingress
annotations:
nginx.ingress.kubernetes.io/rewrite-target: /$1
spec:
rules:
- host: api.travel-planner.com
http:
paths:
- path: /itinerary/?(.*)
pathType: Prefix
backend:
service:
name: itinerary-service
port:
number: 80
代码12:Kubernetes部署配置示例
17. 未来发展方向
17.1 技术演进路线
- AI深度集成
-
- 基于深度学习的景点推荐
- 自然语言处理的智能客服
- 图像识别的景点搜索
- 增强现实体验
-
- AR实景导航
- 历史场景重现
- 虚拟导游
- 区块链应用
-
- 去中心化的行程共享
- 不可篡改的评价系统
- 智能合约支付
- 物联网整合
-
- 智能酒店房间控制
- 景点人流监控
- 交通卡口数据整合
17.2 生态扩展计划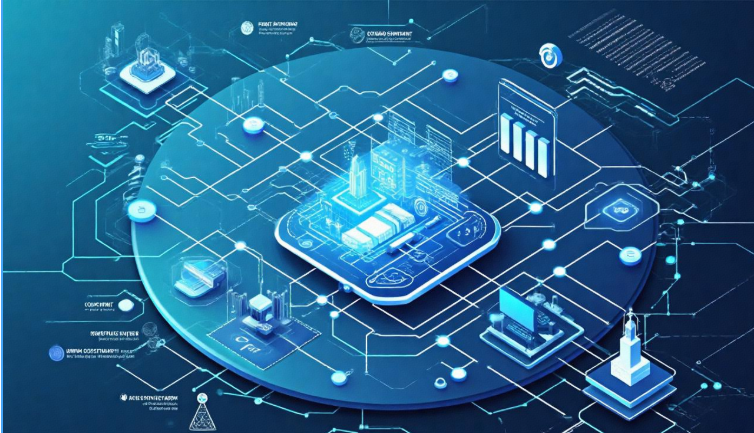
图7:MCP技术生态系统规划
18. 完整系统API参考
18.1 主要API端点
端点 | 方法 | 描述 | 参数 |
| POST | 创建新行程 |
|
| GET | 获取行程详情 | - |
| POST | 优化行程路线 |
|
| GET | 搜索景点 |
|
| GET | 获取推荐景点 |
|
| WebSocket | 实时协作连接 | - |
表2:主要API端点参考
18.2 API响应示例
// 行程详情响应
{
"id": "itn_123456",
"title": "北京三日游",
"days": [
{
"date": "2023-10-01",
"attractions": [
{
"id": "attr_789",
"name": "故宫",
"position": { "lng": 116.397, "lat": 39.918 },
"timeRequired": 4,
"travelTimeFromPrevious": 30
}
],
"travelTime": 120,
"leisureTime": 90
}
],
"stats": {
"totalAttractions": 12,
"