备忘录模式
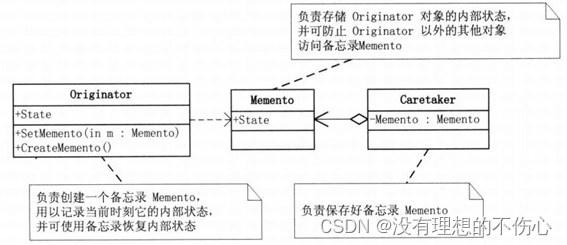
C++
#include <iostream>
#include <string>
using namespace std;
class RoleStateMemento
{
private:
int m_vit;
int m_atk;
int m_def;
public:
RoleStateMemento(int vit, int atk, int def) : m_vit(vit), m_atk(atk), m_def(def) {}
void setVitality(int vit) { m_vit = vit; }
int getVitality() { return m_vit; }
void setAttack(int atk) { m_atk = atk; }
int getAttack() { return m_atk; }
void setDefense(int def) { m_def = def; }
int getDefense() { return m_def; }
};
class GameRole
{
private:
int m_vit;
int m_atk;
int m_def;
public:
RoleStateMemento *SaveState()
{
return (new RoleStateMemento(m_vit, m_atk, m_def));
}
void RecoveryState(RoleStateMemento *memento)
{
m_vit = memento->getVitality();
m_atk = memento->getAttack();
m_def = memento->getDefense();
}
void GetInitState()
{
m_atk = m_def = m_vit = 100;
}
void Fight()
{
m_atk = m_def = m_vit = 0;
}
void StateDisplay()
{
cout << "角色当前状态:" << endl;
cout << "体力:" << m_vit << " "
<< "攻击力:" << m_atk << " "
<< "防御力:" << m_def << endl;
}
};
class RoleStateCaretaker
{
private:
RoleStateMemento *m_memento;
public:
~RoleStateCaretaker()
{
delete m_memento;
}
void setMemento(RoleStateMemento *memento)
{
m_memento = memento;
}
RoleStateMemento *getMemento()
{
return m_memento;
}
};
int main()
{
GameRole *lixiaoyao = new GameRole;
lixiaoyao->GetInitState();
lixiaoyao->StateDisplay();
cout << "\n大战Boss中..." << endl;
RoleStateCaretaker *stateAdmin = new RoleStateCaretaker;
stateAdmin->setMemento(lixiaoyao->SaveState());
lixiaoyao->Fight();
lixiaoyao->StateDisplay();
cout << "\n大战Boss后,恢复进度..." << endl;
lixiaoyao->RecoveryState(stateAdmin->getMemento());
lixiaoyao->StateDisplay();
delete lixiaoyao;
delete stateAdmin;
return 0;
}
C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct
{
int vitality;
int attack;
int defense;
} RoleStateMemento;
RoleStateMemento *create_memento(int vit, int atk, int def)
{
RoleStateMemento *memento = (RoleStateMemento *)malloc(sizeof(RoleStateMemento));
memento->vitality = vit;
memento->attack = atk;
memento->defense = def;
return memento;
}
typedef struct
{
int vitality;
int attack;
int defense;
} GameRole;
void init_game_role(GameRole *role)
{
role->vitality = 100;
role->attack = 100;
role->defense = 100;
}
RoleStateMemento *save_state(GameRole *role)
{
return create_memento(role->vitality, role->attack, role->defense);
}
void restore_state(GameRole *role, RoleStateMemento *memento)
{
role->vitality = memento->vitality;
role->attack = memento->attack;
role->defense = memento->defense;
}
void display_state(GameRole *role)
{
printf("角色当前状态:\n");
printf("体力:%d 攻击力:%d 防御力:%d\n", role->vitality, role->attack, role->defense);
}
typedef struct
{
RoleStateMemento *memento;
} RoleStateCaretaker;
void caretaker_set_memento(RoleStateCaretaker *caretaker, RoleStateMemento *memento)
{
caretaker->memento = memento;
}
RoleStateMemento *caretaker_get_memento(RoleStateCaretaker *caretaker)
{
return caretaker->memento;
}
void cleanup(GameRole *role, RoleStateCaretaker *caretaker)
{
free(caretaker->memento);
free(caretaker);
}
int main()
{
GameRole lixiaoyao;
RoleStateCaretaker *stateAdmin = (RoleStateCaretaker *)malloc(sizeof(RoleStateCaretaker));
init_game_role(&lixiaoyao);
display_state(&lixiaoyao);
printf("\n大战Boss中...\n");
caretaker_set_memento(stateAdmin, save_state(&lixiaoyao));
lixiaoyao.vitality = lixiaoyao.attack = lixiaoyao.defense = 0;
display_state(&lixiaoyao);
printf("\n大战Boss后,恢复进度...\n");
restore_state(&lixiaoyao, caretaker_get_memento(stateAdmin));
display_state(&lixiaoyao);
cleanup(&lixiaoyao, stateAdmin);
return 0;
}